Singly Linked, Linked List.
Intuition:
Have you ever watched a train? Yes, now imagine that you can traverse only one way from the train. In the sense, you can go from box B-11 to B-12 but not vice versa. This is what singly linked, linked lists are.
You start with a head, the head points to another node, the node contains some data, and the address to another node, so on and so forth.
You can add a new node at the head, at the tail, or somewhere in between.
Linked Lists are way better than, arrays if you are not sure about the amount of the data to be stored and may also need to modify some data, in the sense, add new or delete some data in between the chain.
In array, editing data is no doubt easy, but when it comes to adding data between two blocks or deleting data in a similar situation, it becomes a costly job. In such a situation you'll like to use a linked list, singly linked list or a doubly linked list will depend on the context.
Getting started with the code:
Each node will contain a data variable and a pointer variable to the next node. Besides all this, we need to maintain a head variable to keep track of the head of our Linked List.
To check that our linked list is working fine we need to implement a printall function that will print all the values in the Linked List. Here is the working code:
#include <iostream> #include <string> using namespace std; class Node; Node* head = NULL;
class Node{ private: int data; Node* tail; public: int appendathead(int a){ Node* new_node =new Node(); new_node->data = a; new_node->tail = head; head = new_node; return a; } void printall(){ Node* temp = head; while(temp!=NULL){ cout<<temp->data<<endl; temp = temp->tail; } } };
Understanding the code:
First, what variable is what? The head variable is a pointer, that we will use to keep track of the head of the Linked List, obviously, it is initialized to NULL.
Each Node is represented by an object of the class called Node. The private field of each node contains, the essentials of the Linked List, the data it will contain, and the pointer tail to the next node.
The public field contains the function appendathead and printall function, the appendathead takes to input the data in a variable 'a' and adds it to the head of the linked list.
The process of adding the data to the head of the linked list is a four-step process,
First a new node is created, the tail or the next of the new node is assigned the value contained in the head, the data in the new node is updated, and finally, the head is assigned the address of this node.
Note that you need to assign the value to tail first and then shift head and not vice versa, else you'll end up losing the address of the head of the linked list.
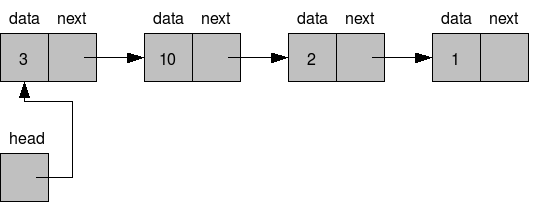
The printall function prints, all the data in the linked list. This is an O(N) process. You first create a temp pointer, you never want to lose the address of the head of the linked list, now you traverse the temp by assigning it the value at its tail, and each time printing the data associated with it. This goes on till temp becomes NULL.
The printall prints the data serial-wise, first of the head and finally at the tail. Thus the result of the above code should be, 7 then 6,10, 20, 30 as we are, each time adding the data at the head, the final head contains the data 7.
The public field contains the function appendathead and printall function, the appendathead takes to input the data in a variable 'a' and adds it to the head of the linked list.
The process of adding the data to the head of the linked list is a four-step process,
First a new node is created, the tail or the next of the new node is assigned the value contained in the head, the data in the new node is updated, and finally, the head is assigned the address of this node.
Note that you need to assign the value to tail first and then shift head and not vice versa, else you'll end up losing the address of the head of the linked list.
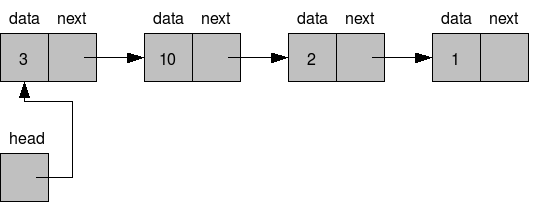
The printall function prints, all the data in the linked list. This is an O(N) process. You first create a temp pointer, you never want to lose the address of the head of the linked list, now you traverse the temp by assigning it the value at its tail, and each time printing the data associated with it. This goes on till temp becomes NULL.
int main(int argc, char const *argv[]) { Node LL =Node(); LL.appendathead(30); LL.appendathead(20); LL.appendathead(10); LL.appendathead(6); LL.appendathead(7); LL.printall(); return 0; }
The printall prints the data serial-wise, first of the head and finally at the tail. Thus the result of the above code should be, 7 then 6,10, 20, 30 as we are, each time adding the data at the head, the final head contains the data 7.
No comments:
Post a Comment